引言
本篇记录最近工作中工程优化的收获,通过优化部门选择器,感觉对树的前中后序遍历理解更深入了;而对编辑器的优化,我发现目前的编辑器像 draftjs、slatejs 等这些比较受欢迎的富文本库对中文的编辑不是那么友好,在解决拼音输入导致页面报错问题的过程中,调试了下 draftjs 0.11.7
的源码,学习了一个新的浏览器 API:MutationObserver,并对 Selection 有了初步的了解
部门算法优化:
源数据大概长这样:
1 | const tree = [ |
算法实现(重点注释):
1 | /** |
Draftjs 拼音输入法报错导致页面白屏
解决方法: 1.在 Draftjs 提供的 Editor 组件外层,包一层 div 2.在这个 div 上绑定 onCompositionStart 这个方法,拼音输入法时会触发 handleCompositionstart 这个函数,在这里先把文本给删除了再把这个编辑器状态更新,可以解决一口气操作拼音输入法的报错问题。
compositionstart是一个 web API,当用户使用拼音输入法开始输入汉字时,这个事件就会被触发
1 | handleCompositionstart = () => { |
就目前的调查来看,目前 0.11.7 中,Draftjs 对拼音的输入是通过 MutationObserver 这个 API 来监控文档变化的,然后会在这里处理选区Selection相关收集和操作。但是升级到最新的 0.11.7 版本,发现这个版本对拼音输入法的修改收集可能存在问题,具体是哪里的问题,我不确定也不会改,降级到 0.10.5 版本,发现 0.11.7 这里的问题都不存在了,所以,最终,我选择将 Daraftjs 升级到 0.10.5,加上上面的 onCompositionStart 方法解决了报错白屏的问题。
但是升级的同时,Daraftjs 内部本身会报错,这是由于之前我们的版本是 V8,V10 的版本对一些 API 想要废弃,所以在开发环境出现一堆 warning

一开始搜DraftEntity.get
,项目里并没有搜到结果,后面仔细看发现/draft-js/src/Draft.js 这里的导出重命名了
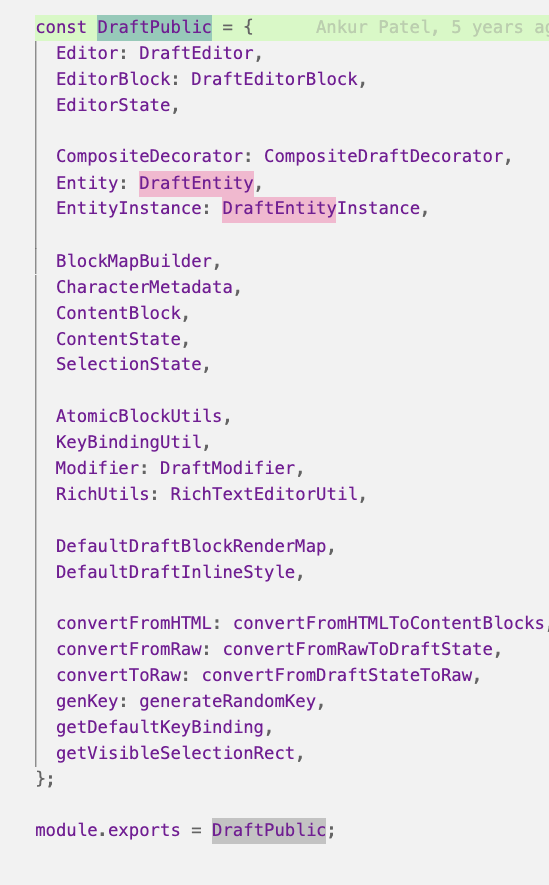
所以导致我搜索关键字错误了,实际上,就是所有用到 Entity.get 的调用都需要升级,升级后,warning 就都解决了。
MutationObserver 用法
1 | function callback(mutationList, observer) { |
因为不熟悉 MutationObserver 的使用,在搜索文章的过程中,发现给文档加水印时,为了不让用户把水印隐藏,刚好这个方法能派上用场,web 页面水印传送门